2.15. Lecture 14: Agent-based models¶
Before this class you should:
Read Think Complexity, Chapter 9
Before next class you should:
Read Think Complexity, Chapter 10
Note Taker: Syed Haadi Rehan
Introduction to Agent-Based Models: - They involve systems governed by simple rules, similar to Cellular Automata (CA). Like CAs, they can be considered “rule-based”, however, ABMs differ from CAs because they include entities called “agents” that can have different rules. In contrast, the components in previously studied models were identical.
Agents are intended to model people and other entities. They typically:
Gather information about the world.
Make decisions based on this information and their internal rules.
Take actions within the environment.
Agents are usually situated in space or within a network and interact with each other locally and usually have imperfect, local information about their surroundings.
Key Concepts in Agent Based Models:
Agents are autonomous, individual entities with dynamic, adaptive behaviors and interact with each other and with their environment.
These interactions can lead to emergent outcomes at the system level, that are not explicitly programmed into the individual agents. They can be surprising and difficult to predict even when the individual agent rules are known.
ABMs are particularly useful for understanding the relationships between individual decisions and overall system behavior.
The environment plays a crucial role in ABMs. It can be explicitly represented and can be dynamic. Agents are situated within this environment and interact with it and each other for continuous feedback.
Schelling’s Model of Segregation:
This model explores how individual preferences for living near similar neighbors can lead to large-scale segregation, even if individuals are not strongly biased.
The Schelling model operates on a grid where agents of different types (e.g., red and blue) and empty spaces are located. Each agent has a threshold (p) representing the minimum fraction of similar neighbors they require to be “happy”. If an agent is unhappy (fewer than p similar neighbors), it moves to a randomly chosen empty location on the grid. The simulation continues until a stable state is reached where no agents are unhappy.
Even with relatively low preferences for same-type neighbors (e.g., p=0.3), the model demonstrates a significant degree of segregation emerges. This suggests that segregation can arise from individual preferences without necessarily implying strong racism.
2.15.1. Implementation of Schelling and Sugarscape Simulations¶
class Schelling(Cell2D):
def __init__(self, n, p):
self.p = p
# 0 is empty, 1 is red, 2 is blue
choices = np.array([0, 1, 2], dtype=np.int8)
probs = [0.1, 0.45, 0.45]
self.array = np.random.choice(choices, (n, n), p=probs)
a = self.array
red = a == 1
blue = a == 2
empty = a == 0
options = dict(mode='same', boundary='wrap')
kernel = np.array([
[1, 1, 1],
[1, 0, 1],
[1, 1, 1]
], dtype=np.int8)
num_red = correlate2d(red, kernel, **options)
num_blue = correlate2d(blue, kernel, **options)
num_neighbors = num_red + num_blue
frac_red = num_red / num_neighbors
frac_blue = num_blue / num_neighbors
frac_same = np.where(red, frac_red, frac_blue)
frac_same[empty] = np.nan
The Schelling class inherits from Cell2D, indicating it’s a grid-based model. The step function involves identifying unhappy agents, finding empty locations, and moving the unhappy agents to a random empty spot.
2.15.2. Sugarscape Model¶
This model simulates a simple economy where agents move on a 2D grid to harvest and accumulate “sugar,” representing wealth.
Each agent has individual attributes such as:
Initial Sugar: A starting amount of wealth
Metabolism: The amount of sugar consumed per time step
Vision: The distance an agent can “see” to locate sugar
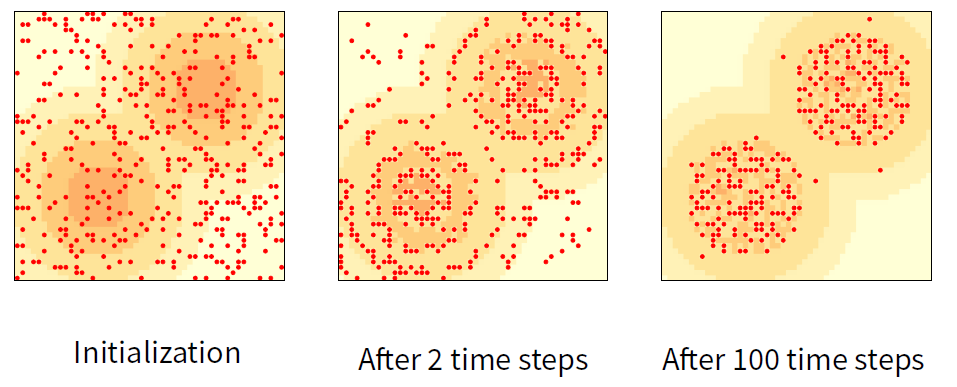
Agents use their vision to find nearby cells with sugar and move to the location with the most sugar within their visual range. After moving, agents “harvest” the sugar from their current location, which depletes the sugar in that cell temporarily. If sugar level drops to zero, the agent “dies”.
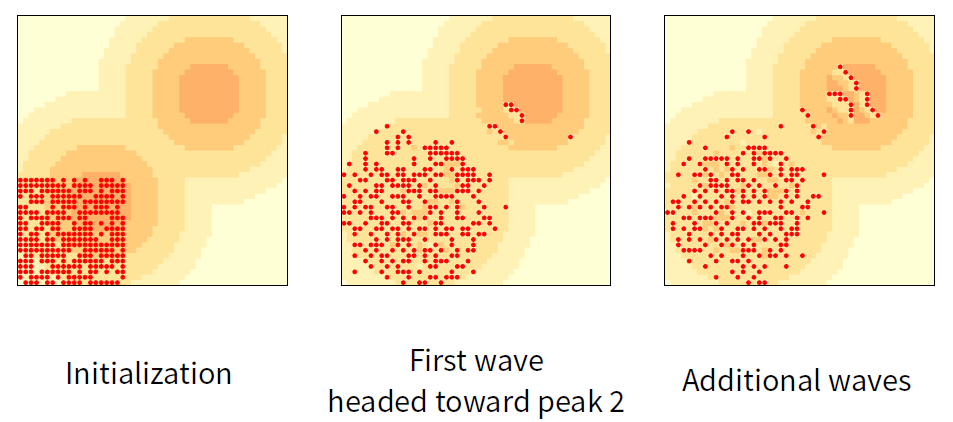
Simulations of Sugarscape often show the emergence of wealth inequality among agents, even though they start with randomly chosen attributes and follow relatively simple rules.
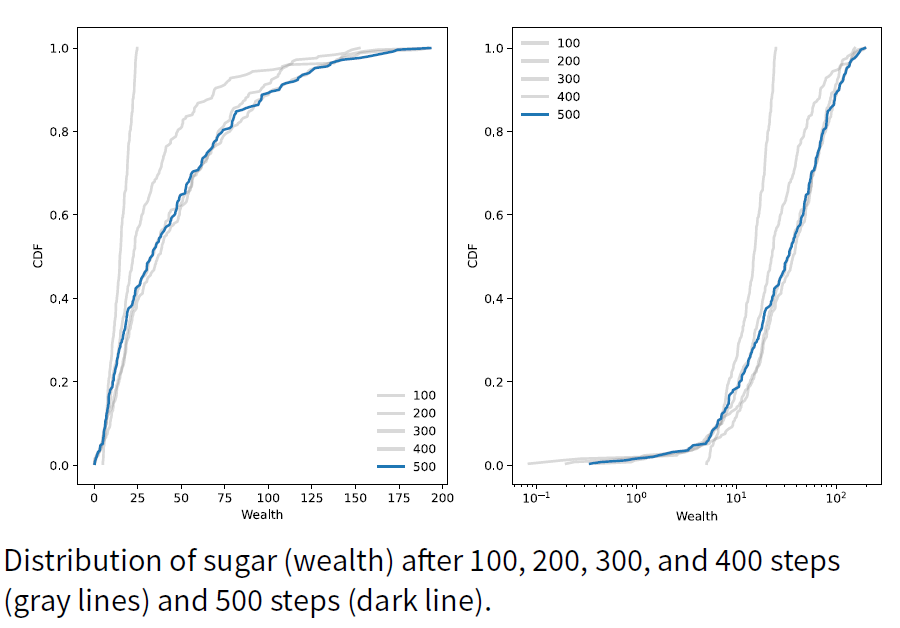